Dark Mode
Launch Express comes with built-in support for dark mode, allowing you to create a visually appealing and accessible user interface that adapts to user preferences and system settings.
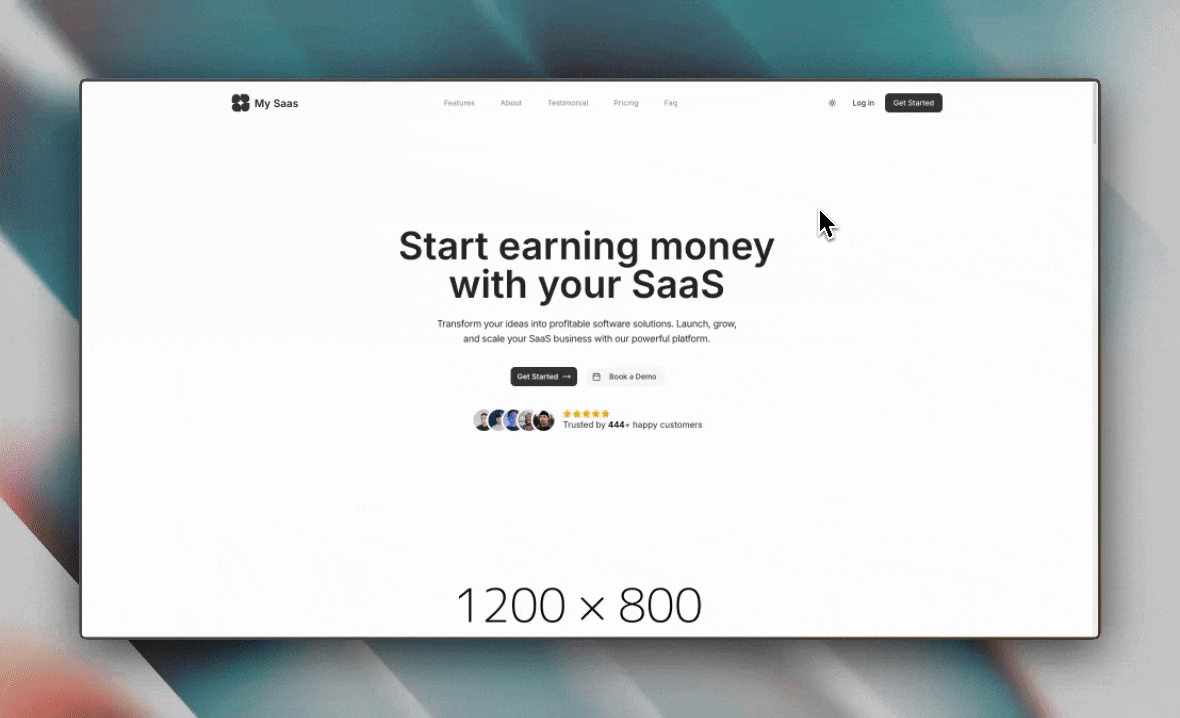
You can preview how Launch Express components look in both light and dark modes right here in the documentation. Simply use the theme toggle in the documentation’s navigation bar to switch between light and dark modes.
Overview
The dark mode feature in Launch Express leverages the power of Tailwind CSS and the next-themes
package to provide a seamless dark mode experience. Users can switch between light, dark, and system-based themes.
Configuration
Initial Theme Setting
You can set the initial theme for your application in the config.ts
file:
Available options are:
'light'
: Always use light mode'dark'
: Always use dark mode'system'
: Use the system preference (default if not specified)
Implementation
Theme Provider
Launch Express wraps your application with a ThemeProvider
component from next-themes
. This provider is typically set up in the root layout file:
Dark Mode Toggle
A dark mode toggle component is included in Launch Express. You can find it in components/DarkModeToggle.tsx
. This component allows users to switch between light and dark modes.
Usage in Components
When creating or styling components, use Tailwind’s dark mode variant to specify different styles for dark mode:
Custom Dark Mode Logic
If you need more control over when dark mode is applied, you can use the useTheme
hook from next-themes
: