Getting Started
Features
- AI Providers
- AI Services
- Authentication
- Database
- Payments
- UI & Design
- Content
- Analytics & SEO
- Marketing & Growth
Subscriptions
The Subscription pricing configuration allows you to set up and manage recurring payment plans for your application. This configuration is defined in the subscription-pricing.ts file and can be utilized through the SubscriptionPricing component.
The configuration in subscription-pricing.ts
consists of the following settings for each plan:
Go to the Stripe Dashboard to create a new subscription for each plan. The price identifier is used to identify the plan in the application. The price identifier is unique to each plan and can be found in the Stripe Dashboard.
Then copy the price identifier and paste it into the monthly
and annually
fields of the priceId
in the subscription-pricing.ts
file.
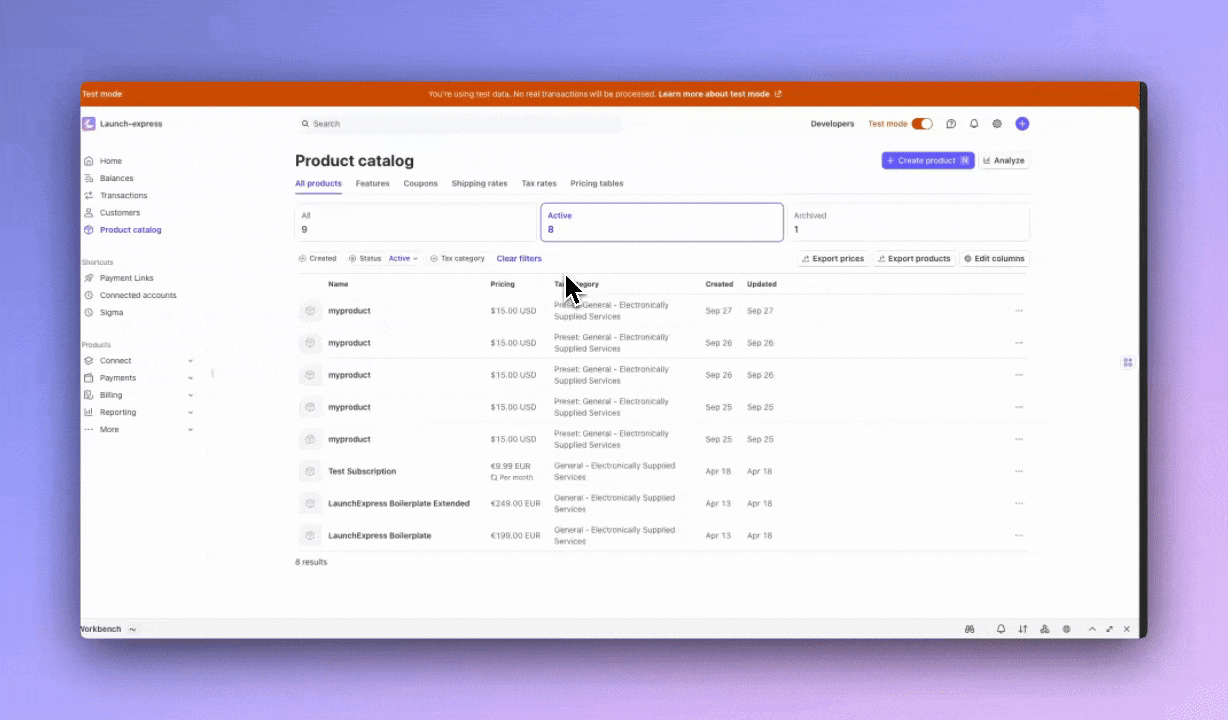
export const Subscriptions: SubscriptionConfig = {
display: {
showAnnualToggle: true,
highlightAnnualSavings: true,
currency: 'USD',
showTrialDays: true,
},
plans: [
{
name: 'Pro', // Display name of the pricing plan
id: 'pro', // Unique identifier for the plan
type: 'trial', // The type of plan (trial, paid, free or enterprise)
trialDays: 14, // The number of trial days
description: 'Best for growing teams', // The description of the plan
isMostPopular: true, // Boolean flag to highlight the most popular plan
pricing: {
monthly: {
amount: 40, // The regular monthly price of the plan
priceId: process.env.NODE_ENV === 'development'
? 'price_123456' // The price identifier to test in the development environment
: 'price_789101', // The price identifier to test in the development environment
discount: {
amount: 32, // The discounted monthly price of the plan
priceId: process.env.NODE_ENV === 'development'
? 'price_123456' // The price identifier to test in the development environment
: 'price_789101', // The price identifier to test in the development environment
percentage: 20, // The percentage discount of the plan
label: 'Limited Time Offer', // The label of the discount
},
},
annually: {
amount: 400, // The regular annual price of the plan
priceId: process.env.NODE_ENV === 'development'
? 'price_123456' // The price identifier to test in the development environment
: 'price_789101', // The price identifier to test in the development environment
bonus: {
amount: 80, // The bonus amount of the plan
description: '2 months free', // The description of the bonus
},
discount: {
amount: 320, // The discounted annual price of the plan
priceId: process.env.NODE_ENV === 'development'
? 'price_123456' // The price identifier to test in the development environment
: 'price_789101', // The price identifier to test in the development environment
percentage: 20, // The percentage discount of the plan
label: 'Limited Time Offer', // The label of the discount
},
},
},
features: [
{ name: 'Feature 1', available: true }, // Array of features included in the plan
{ name: 'Feature 2', available: true },
{ name: 'Feature 3', available: true },
{ name: 'Feature 4', available: true },
{ name: 'Feature 5', available: true },
],
},
...
],
};
Go to the Stripe Dashboard to create a new subscription for each plan. The price identifier is used to identify the plan in the application. The price identifier is unique to each plan and can be found in the Stripe Dashboard.
Then copy the price identifier and paste it into the monthly
and annually
fields of the priceId
in the subscription-pricing.ts
file.
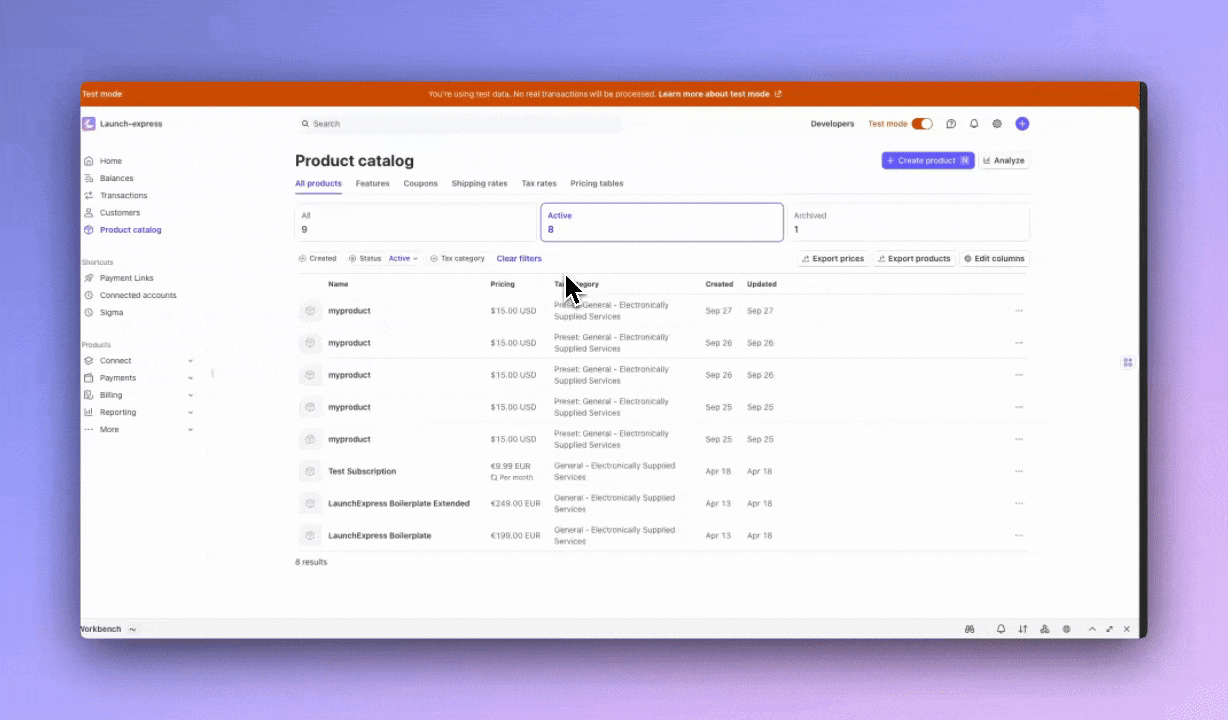
export const Subscriptions: SubscriptionConfig = {
display: {
showAnnualToggle: true,
highlightAnnualSavings: true,
currency: 'USD',
showTrialDays: true,
},
plans: [
{
name: 'Pro', // Display name of the pricing plan
id: 'pro', // Unique identifier for the plan
type: 'trial', // The type of plan (trial, paid, free or enterprise)
trialDays: 14, // The number of trial days
description: 'Best for growing teams', // The description of the plan
isMostPopular: true, // Boolean flag to highlight the most popular plan
pricing: {
monthly: {
amount: 40, // The regular monthly price of the plan
priceId: process.env.NODE_ENV === 'development'
? 'price_123456' // The price identifier to test in the development environment
: 'price_789101', // The price identifier to test in the development environment
discount: {
amount: 32, // The discounted monthly price of the plan
priceId: process.env.NODE_ENV === 'development'
? 'price_123456' // The price identifier to test in the development environment
: 'price_789101', // The price identifier to test in the development environment
percentage: 20, // The percentage discount of the plan
label: 'Limited Time Offer', // The label of the discount
},
},
annually: {
amount: 400, // The regular annual price of the plan
priceId: process.env.NODE_ENV === 'development'
? 'price_123456' // The price identifier to test in the development environment
: 'price_789101', // The price identifier to test in the development environment
bonus: {
amount: 80, // The bonus amount of the plan
description: '2 months free', // The description of the bonus
},
discount: {
amount: 320, // The discounted annual price of the plan
priceId: process.env.NODE_ENV === 'development'
? 'price_123456' // The price identifier to test in the development environment
: 'price_789101', // The price identifier to test in the development environment
percentage: 20, // The percentage discount of the plan
label: 'Limited Time Offer', // The label of the discount
},
},
},
features: [
{ name: 'Feature 1', available: true }, // Array of features included in the plan
{ name: 'Feature 2', available: true },
{ name: 'Feature 3', available: true },
{ name: 'Feature 4', available: true },
{ name: 'Feature 5', available: true },
],
},
...
],
};
Go to the Lemonsqueezy Dashboard to create a new price for each subscription plan. The price identifier is used to identify the plan in the application. The price identifier is unique to each plan and can be found in the Lemonsqueezy Dashboard.
Then copy the price identifier and paste it into the monthly
and annually
fields of the priceId
in the subscription-pricing.ts
file.
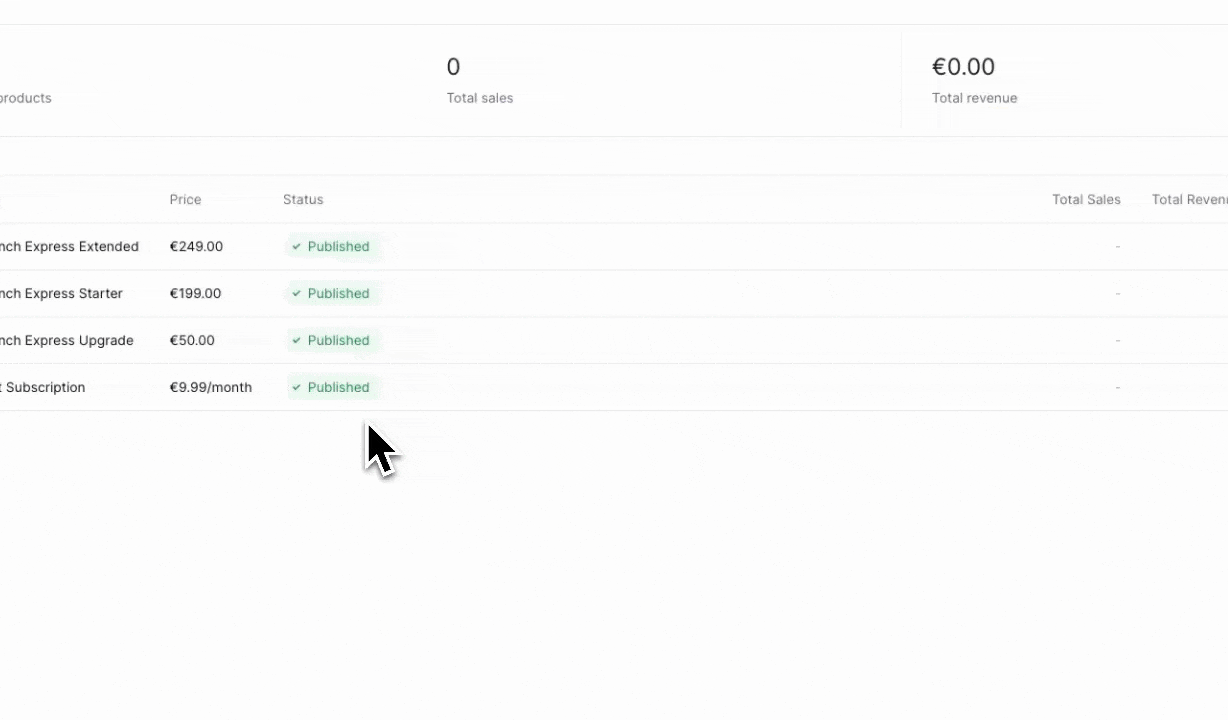
export const Subscriptions: SubscriptionConfig = {
display: {
showAnnualToggle: true,
highlightAnnualSavings: true,
currency: 'USD',
showTrialDays: true,
},
plans: [
{
name: 'Pro', // Display name of the pricing plan
id: 'pro', // Unique identifier for the plan
type: 'trial', // The type of plan (trial, paid, free or enterprise)
trialDays: 14, // The number of trial days
description: 'Best for growing teams', // The description of the plan
isMostPopular: true, // Boolean flag to highlight the most popular plan
pricing: {
monthly: {
amount: 40, // The regular monthly price of the plan
priceId: process.env.NODE_ENV === 'development'
? '123456' // The price identifier to test in the development environment
: '789101', // The price identifier to test in the development environment
discount: {
amount: 32, // The discounted monthly price of the plan
priceId: process.env.NODE_ENV === 'development'
? '123456' // The price identifier to test in the development environment
: '789101', // The price identifier to test in the development environment
percentage: 20, // The percentage discount of the plan
label: 'Limited Time Offer', // The label of the discount
},
},
annually: {
amount: 400, // The regular annual price of the plan
priceId: process.env.NODE_ENV === 'development'
? '123456' // The price identifier to test in the development environment
: '789101', // The price identifier to test in the development environment
bonus: {
amount: 80, // The bonus amount of the plan
description: '2 months free', // The description of the bonus
},
discount: {
amount: 320, // The discounted annual price of the plan
priceId: process.env.NODE_ENV === 'development'
? '123456' // The price identifier to test in the development environment
: '789101', // The price identifier to test in the development environment
percentage: 20, // The percentage discount of the plan
label: 'Limited Time Offer', // The label of the discount
},
},
},
features: [
{ name: 'Feature 1', available: true }, // Array of features included in the plan
{ name: 'Feature 2', available: true },
{ name: 'Feature 3', available: true },
{ name: 'Feature 4', available: true },
{ name: 'Feature 5', available: true },
],
},
...
],
};
The SubscriptionPricing component is used to display the one-time payment plans in the application.
Was this page helpful?